Artificial Intelligence (AI) is reshaping industries across the globe, and software development is no exception.…
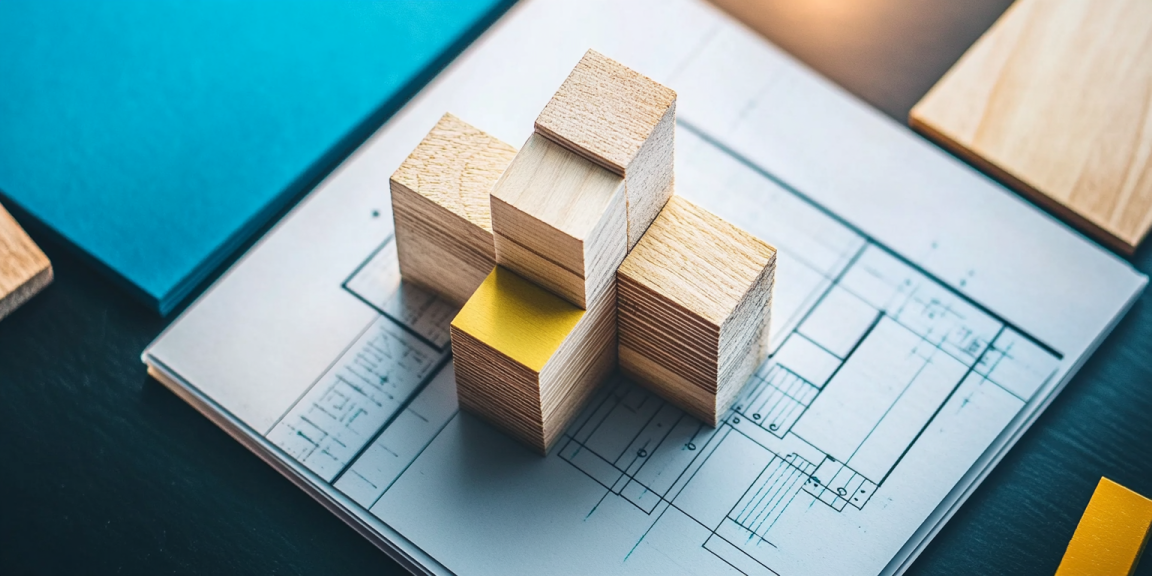
Laravel is one of the most popular PHP frameworks used by developers to build robust, scalable, and secure web applications. With its elegant syntax and powerful features, Laravel simplifies many of the complexities of web development, making it a go-to choice for both beginners and experienced developers. In this article, we will guide you through the steps on how to start your project using the Laravel framework, focusing on the preparation, planning, and setup phases rather than diving into specific code examples.
Why Choose Laravel?
Before jumping into the process of starting a project, it’s important to understand why Laravel has gained such popularity in the web development community.
1. Simple and Elegant Syntax
Laravel is known for its clean, readable syntax that makes it easy to write and maintain code. Its design patterns are intuitive, and the framework comes with built-in functionalities that save time during development. Whether you’re building a small website or a large-scale application, Laravel offers the tools to make the process easier.
2. MVC Architecture
Laravel follows the Model-View-Controller (MVC) architectural pattern, which is great for separating concerns within your application. This separation ensures that your application is organized, making it easier to manage, test, and maintain.
3. Comprehensive Documentation
Laravel’s extensive documentation makes it beginner-friendly and helps developers of all skill levels. Whether you’re encountering a problem or learning a new feature, the Laravel documentation offers in-depth guides and explanations to help you navigate through any challenges.
4. Community Support
Laravel has a large and vibrant community. With thousands of developers contributing to the framework, you’ll always find solutions to your issues and the latest trends in web development. The Laravel ecosystem also includes tools like Forge, Envoyer, and Nova, which further streamline the development process.
5. Security Features
Security is a top concern in web development. Laravel comes with built-in tools to protect your application from common vulnerabilities such as SQL injection, cross-site request forgery (CSRF), and cross-site scripting (XSS).
Steps to Start Your Project Using Laravel
Now that we have a basic understanding of why Laravel is so popular, let’s look at how to start a project with the framework.
Step 1: Define Your Project Goals
Before you start coding, it’s essential to have a clear understanding of what your project is about. Take time to define the objectives of your project, the core features, the target audience, and any unique functionality that your application will offer. This step is crucial as it will guide the structure of your application, determine what features you’ll need, and help you plan your workflow.
Step 2: Install Laravel
Once you have a clear plan for your project, the next step is to install Laravel on your development environment.
Setting up Your Development Environment:
To start using Laravel, you’ll need a PHP development environment. You can either use a local server like XAMPP, WAMP, or Docker, or opt for cloud-based environments like Laravel Forge.
- Install Composer: Composer is a dependency management tool for PHP that helps you manage the packages Laravel relies on. It’s essential for Laravel projects. You can download and install Composer from getcomposer.org.
- Install Laravel via Composer: After installing Composer, open your terminal or command prompt and run the following command to create a new Laravel project:
- Set Up a Local Server: If you’re using a local development environment, make sure your PHP server is running. If you’re using Laravel’s built-in server, you can run:
- Check Your Installation: Once your environment is set up, navigate to
http://localhost:8000
in your web browser, and you should see the default Laravel welcome page.
Step 3: Set Up the Database
Most web applications need a database to store data. Laravel supports a variety of database management systems such as MySQL, PostgreSQL, SQLite, and SQL Server.
- Create a Database: Before connecting Laravel to a database, you’ll need to create a database on your chosen server.
- Configure .env File: Laravel stores sensitive information, such as your database credentials, in the
.env
file located in the root directory of your project. Open the.env
file and add your database connection details.Example for MySQL: - Run Migrations: Laravel has a built-in migration system that allows you to define and modify your database schema in a version-controlled manner. You can create migration files using Artisan, Laravel’s command-line interface, and apply them with the following command:
This will create the necessary tables in your database according to the migration definitions.
Step 4: Define Routes and Controllers
In Laravel, routes handle the incoming requests, and controllers contain the logic for responding to those requests.
- Define Routes: Open the
routes/web.php
file, where you can define the HTTP routes for your application. Laravel’s routing system is intuitive, allowing you to easily create routes that respond to different HTTP methods (GET, POST, PUT, DELETE, etc.). - Create Controllers: After defining your routes, you will need to create controllers to handle the logic. Laravel provides a simple Artisan command to generate controllers:
Once the controller is created, you can link it to a route and define methods to handle the requests.
Step 5: Build Your Views with Blade
Laravel uses Blade, a powerful templating engine, to render views. Blade allows you to create clean and reusable views by combining plain PHP with HTML.
- Create Views: Views are stored in the
resources/views
directory. You can create a Blade view by simply creating a.blade.php
file. For example, you might create ahome.blade.php
file for the homepage. - Passing Data to Views: You can pass data to your views using the
with()
method or an associative array. For example: - Using Blade Syntax: Blade provides a range of features, such as conditional statements, loops, and template inheritance, to help you build dynamic pages with minimal effort.
Step 6: Implement Authentication (Optional)
If your project requires user authentication, Laravel comes with built-in authentication features, including registration, login, and password reset functionality.
- Install Laravel Breeze, Jetstream, or Fortify: Laravel offers various authentication packages to suit different needs. You can install one of these packages to quickly set up user authentication.
- Run the Authentication Scaffold: After installing your chosen authentication package, you can use Artisan to generate the necessary views and routes.
Step 7: Test Your Application
Testing is an important part of any development process, and Laravel makes it easy to write unit tests and feature tests to ensure your application works as expected.
- Run Tests: Laravel comes with PHPUnit, a testing framework that allows you to write tests for your application. You can run your tests using the following command:
- Debugging: Use Laravel’s built-in error handling and logging tools to debug and troubleshoot any issues during development.
Conclusion
Starting a project with the Laravel framework is a straightforward process that involves careful planning, setting up the development environment, and leveraging Laravel’s powerful features. Whether you’re building a small web application or a large enterprise solution, Laravel provides the tools you need to develop efficiently and securely. By following the steps outlined above, you’ll be able to kickstart your project, ensuring a solid foundation for growth and scalability.