Artificial Intelligence (AI) is reshaping industries across the globe, and software development is no exception.…
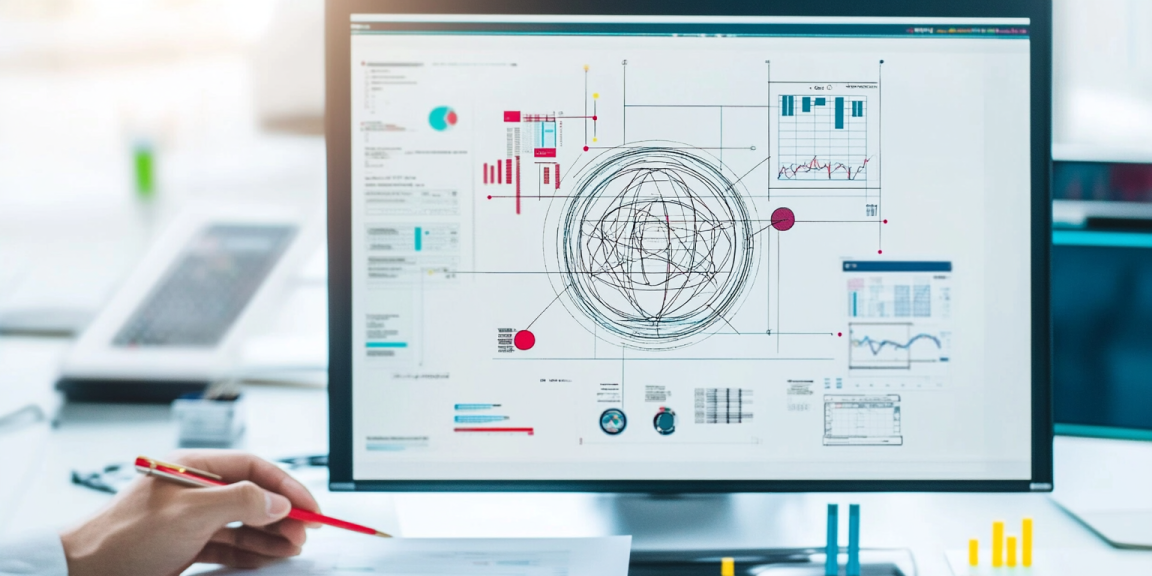
In recent years, React.js has become one of the most popular JavaScript libraries for building user interfaces, particularly for single-page applications. Its flexibility, ease of integration, and vast ecosystem make it a favorite choice for developers around the world. Whether you’re a beginner or a seasoned developer, React offers tools and features that can help you build efficient, scalable, and interactive web applications. In this guide, we’ll walk you through how to start a project using React.js, from setting up your development environment to building your first component.
What is React.js?
React.js, commonly referred to as React, is an open-source JavaScript library developed by Facebook. It allows developers to build user interfaces (UIs) that are both fast and interactive. React follows a component-based architecture, meaning that UIs are built by composing small, reusable components, each responsible for rendering a specific piece of the interface. This makes React highly modular and easy to maintain.
Unlike other JavaScript frameworks that handle both the view and the business logic, React is primarily focused on the view layer. It can be integrated with other libraries and frameworks, such as Redux for state management or React Router for routing, to form a complete development stack.
Why Use React.js?
Before diving into the specifics of setting up a React project, it’s important to understand why React is so widely used:
- Component-Based Architecture: React’s component-based structure promotes reusable code, making it easier to maintain and scale applications.
- Virtual DOM: React uses a virtual DOM to optimize the rendering process, which increases the performance of applications.
- Rich Ecosystem and Libraries: React has a large ecosystem of tools and libraries, including React Router, Redux, and Next.js, that enhance its capabilities.
- Declarative Syntax: React’s declarative syntax allows developers to describe how the UI should look based on the state, making the code more intuitive and easier to debug.
- Strong Community Support: React has a large and active community, ensuring that developers have access to plenty of tutorials, documentation, and support.
Now that we’ve discussed the benefits of using React, let’s walk through the steps to get started with your first React project.
1. Setting Up the Development Environment
Before you can start building with React, you need to set up your development environment. This involves installing Node.js and the React development tools.
Step 1: Install Node.js
React.js requires Node.js for its build tools and package management system (npm). To get started, download and install the latest version of Node.js from the official website: Node.js Download.
Once installed, verify the installation by running the following command in your terminal or command prompt:
bash
node -v
npm -v
This will display the version of Node.js and npm (Node Package Manager) installed on your system.
Step 2: Install the React Development Tools
React comes with a command-line tool called create-react-app
that automates the process of setting up a React project. This tool configures everything you need to start building, including the project structure, build tools, and dependencies.
To install create-react-app
, run the following command:
bash
npm install -g create-react-app
Once installed, you can create a new React project using the following command:
bash
npx create-react-app my-first-react-app
This will create a new folder called my-first-react-app
with all the necessary files and folder structure.
Step 3: Start the Development Server
Navigate to the newly created project folder:
bash
cd my-first-react-app
Then, run the development server:
bash
npm start
This will start a local development server and open the default React app in your web browser, typically at http://localhost:3000
. Now, you have a working React application running locally, and you’re ready to begin development!
2. Understanding the Project Structure
When you create a new React project using create-react-app
, you’ll see several folders and files. Here’s a breakdown of the key files and folders you’ll be working with:
- public/index.html: The main HTML file for your project. React will render your components inside the
div
with the idroot
in this file. - src/index.js: The entry point for your React application. This is where React gets rendered into the
root
div in the HTML file. - src/App.js: The default component provided by
create-react-app
. You’ll modify this file to add your own components and logic. - src/App.css: The default CSS file where you can style your components.
React uses JavaScript (or TypeScript) and CSS for styling, so you won’t need to deal with complex build configurations initially. create-react-app
takes care of all that for you.
3. Building Your First Component
React applications are built using components. Components are the building blocks of React and can be thought of as reusable pieces of code that define a part of the user interface. Let’s start by creating a simple component.
Step 1: Modify the App.js
Component
In the src
folder, open the App.js
file. By default, this file contains some boilerplate code. You can delete everything inside the App.js
file and replace it with a simple functional component.
Here’s an example of a basic React component:
javascript
import React from 'react';
import './App.css';
function App() {return (
<div className=“App”>
<h1>Hello, React!</h1>
<p>Welcome to your first React project.</p>
</div>
);
}
export default App;
Step 2: Add Styling to the Component
React supports CSS styling, and the default setup includes an App.css
file for styling. You can modify this file to add your own styles for the components.
For example, you can add the following CSS to center the text and style the background color:
css
.App {
text-align: center;
background-color: #f0f0f0;
padding: 20px;
}
h1 {color: #333;
}
This will style the App
component with a centered layout and a light background.
Step 3: Save and View the Changes
After making these changes, save the file. Since the development server is running, the changes will automatically reflect in the browser.
4. Using State and Props in React
One of the key features of React is the ability to manage state and pass data between components using props. Let’s modify the App.js
file to demonstrate these concepts.
Step 1: Add State to the Component
React components can have state, which allows them to hold data that changes over time. Here’s how you can add a piece of state to the App
component using the useState
hook:
javascript
import React, { useState } from 'react';
import './App.css';
function App() {const [count, setCount] = useState(0);
return (
<div className=“App”>
<h1>Hello, React!</h1>
<p>Welcome to your first React project.</p>
<button onClick={() => setCount(count + 1)}>
Clicked {count} times
</button>
</div>
);
}
export default App;
In this example, we use the useState
hook to create a state variable called count
. The setCount
function updates the state whenever the button is clicked.
Step 2: Pass Props to a Child Component
Now, let’s create a child component that accepts props. Create a new file Greeting.js
inside the src
folder and add the following code:
javascript
import React from 'react';
function Greeting(props) {
return <h2>{props.message}</h2>;
}
export default Greeting;
Next, import and use this Greeting
component in the App.js
file:
javascript
import React, { useState } from 'react';
import './App.css';
import Greeting from './Greeting';
function App() {const [count, setCount] = useState(0);
return (
<div className=“App”>
<h1>Hello, React!</h1>
<Greeting message=“This is a message from the parent component.” />
<button onClick={() => setCount(count + 1)}>
Clicked {count} times
</button>
</div>
);
}
export default App;
Here, we pass a prop called message
to the Greeting
component, which will display the message passed from the parent component.
5. Conclusion
Starting a project with React.js is straightforward, especially with tools like create-react-app
that automate much of the setup process. By following the steps outlined above, you can quickly set up your development environment, build your first component, and use React’s powerful features like state and props to make your application dynamic.
React is not just a library, but an entire ecosystem with a thriving community and endless resources for learning and support. Whether you’re building a small personal project or a large-scale enterprise application, React can help you create fast, scalable, and maintainable user interfaces. As you become more familiar with React, you can dive deeper into its advanced features and integrate other tools and libraries to enhance your project.
With React’s simplicity and flexibility, you can quickly start building web applications that users love, ensuring a successful start to your journey as a React developer.