Artificial Intelligence (AI) is reshaping industries across the globe, and software development is no exception.…
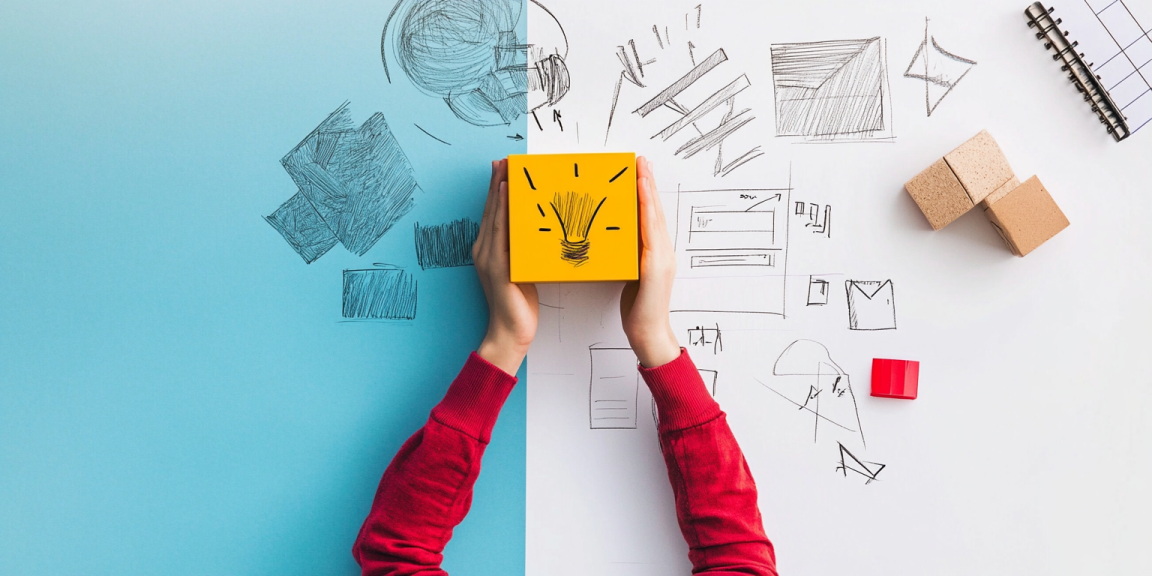
Programming errors, or “bugs,” are an inevitable part of development. They range from simple syntax issues to complex runtime problems, and effectively addressing them is a crucial skill for any programmer. In this guide, we’ll explore the most common types of programming errors, how to identify them, and best practices to fix and prevent them.
Understanding Programming Errors
Before diving into solutions, it’s important to classify errors to understand their nature better. Here are the primary categories of programming errors:
- Syntax Errors
These occur when the code violates the rules of the programming language. Examples include missing semicolons, misspelled keywords, or unmatched brackets. - Runtime Errors
These happen when the program is executed. Examples include dividing by zero, accessing an invalid index, or null pointer exceptions. - Logical Errors
These are the trickiest to detect. The program runs without crashing but produces incorrect results due to flawed logic or calculations. - Semantic Errors
Occur when the program is syntactically correct but does not align with the intended meaning. - Compilation Errors
Specific to compiled languages like Java or C++, these arise during the compilation process, often due to syntax or type mismatches.
How to Identify Programming Errors
- Use Debuggers
Integrated development environments (IDEs) like Visual Studio, Eclipse, or PyCharm come equipped with powerful debugging tools to identify errors. - Read Error Messages Carefully
Most programming environments provide detailed error messages. Learn to interpret them to pinpoint the root cause. - Check the Logs
Logs are invaluable for identifying runtime and logical errors. Add logging to your code to trace the flow of execution and locate issues. - Reproduce the Issue
Consistently reproducing the error helps isolate the conditions that cause it. - Break the Problem Down
Divide your code into smaller segments and test them individually to locate the source of the error.
Common Programming Errors and How to Handle Them
1. Syntax Errors
- Example:
- Cause: Missing closing quotation mark.
- Solution:
Use an IDE with syntax highlighting and error checking. Review your code carefully for typos or missing elements.
2. Null Pointer Exceptions
- Example:
- Cause: Trying to access a property of an object that is
null
.
- Cause: Trying to access a property of an object that is
- Solution:
Always check for null values before accessing object properties or methods. Use defensive programming techniques like:
3. Off-by-One Errors (OBOE)
- Example:
- Cause: Iterating one step too many, leading to an out-of-bounds error.
- Solution:
Ensure loops start and end at the correct indices. Usearray.length - 1
when necessary.
4. Infinite Loops
- Example:
- Cause: Loop termination condition is not properly defined.
- Solution:
Always include a clear exit condition orbreak
statement within the loop.
5. Floating-Point Errors
- Example:
- Cause: Precision errors due to how floating-point numbers are represented.
- Solution:
Use libraries or data types that support high-precision arithmetic, likeDecimal
in Python.
6. Mismatched Data Types
- Example:
- Cause: Attempting to assign an incompatible type.
- Solution:
Use proper type conversion or casting methods. Example:
7. Undefined Variables
- Example:
- Cause: Using a variable before declaring it.
- Solution:
Always declare variables before use. Tools like ESLint for JavaScript can catch such issues during development.
8. Incorrect API Calls
- Example: Calling a function with the wrong parameters.
- Solution:
Refer to the official documentation for APIs and libraries to ensure correct usage. Use IDEs that support autocomplete and provide parameter hints.
Best Practices for Handling Errors
- Write Clean Code
- Follow coding standards and conventions.
- Use meaningful variable and function names to make the code self-explanatory.
- Implement Error Handling
- Use
try-catch
blocks for handling runtime errors. - Create custom error messages to help diagnose issues faster.
- Use
- Adopt Defensive Programming
- Anticipate potential errors and handle them proactively. For example, check user inputs before processing.
- Unit Testing
- Write unit tests to verify the correctness of individual code components. Testing frameworks like Jest, JUnit, or PyTest are invaluable.
- Code Reviews
- Collaborate with peers to review code for potential issues before deployment.
- Use Version Control
- Tools like Git help track changes, making it easier to identify when and where an error was introduced.
- Automate Debugging and Testing
- Use tools like Selenium for automated testing and debugging to ensure consistent performance.
Tools to Assist in Debugging and Error Management
- Debugging Tools
- Chrome DevTools: For debugging JavaScript in web applications.
- GDB: A debugger for C and C++ programs.
- Linters and Code Analyzers
- ESLint (JavaScript), Pylint (Python), or SonarQube can detect potential errors during development.
- Logging Libraries
- Log4j (Java), Winston (Node.js), or Python’s
logging
module help track runtime errors.
- Log4j (Java), Winston (Node.js), or Python’s
- Error Monitoring Services
- Tools like Sentry or New Relic provide real-time error tracking in production environments.
How to Prevent Errors in the Future
- Learn from Past Mistakes
Keep an error log and review it periodically to identify recurring issues and address their root causes. - Stay Updated
Programming languages and frameworks evolve. Keep your skills current to avoid outdated practices. - Use Libraries and Frameworks Wisely
Choose well-maintained libraries with active community support. - Optimize for Maintainability
Write modular code with clear documentation to make debugging and updates easier.
Conclusion
Handling programming errors effectively is a blend of technical skills, proactive strategies, and leveraging the right tools. By understanding the types of errors, using systematic debugging approaches, and adhering to best practices, developers can minimize downtime, ensure smooth functionality, and deliver reliable applications.
Errors are a natural part of development, but with the right mindset and methodology, every bug becomes an opportunity to learn and improve. So, embrace the process, and let your debugging skills evolve with your programming journey!